Swarm Simulation
April 2022 - December 2022Create a simulator for swarm using socket programming (Github link)
Overview
The project aims to create a simulator for swarm robots. The project involves implementing the existing API's functionality by creating a custom API that interfaces with the simulator. The project uses socket programming and client - server architecture to launch several instances of the robot as processes, that can communicate with a central server simulator. This central simulator keeps track of time, dynamic model of the robots, and integrates the world. There is also visualization using pygame that occurs in a seperate process.
Download and run
Clone the repository in a folder. Go inside sim_pkg folder. Run python3 coachbot-simulation.py -fn userfile in sim_pkg folder. The user.py file should be in sim_pkg folder. The user file name should be given without .py extension in the above command.
This should launch the simulation with the mentioned number of robots running the algorithm given in the user program. The simulation environment can be set by setting the variables in config.json:
- TIME_ASYNC - 0 to make the robot time synced and 1 to introduce time asynchronous initialization for robots.
- NUMBER_OF_ROBOTS - Number of robots to launch
- COMM_RANGE - The range of communication (in meters)
- PACKET_SUCCESS_PERC - Set the success rate of message packets (values in decimal range from 0 to 1)
- REAL_TIME_FACTOR - Maximum allowable real time factor
- NUM_OF_MSGS - Maximum number of messages to keep in the buffer
- MSG_SIZE - Maximum size of each message in buffer
- WIDTH - width of the arena (vertical axis)
- LENGTH - length of the arena (horizontal axis length)
- USE_INIT_POS - use the initialization python program to initialize position of the robot
- SIM_TIME_STEP - Set the simulation time step for each loop cycle
- USE_VIS - If 1, then visualizer will be used. If 0, then visualizer will not be used and instead all states and the time are logged automatically to sim.log
Structure
The main files include the following:
- simulator.py - The main simulator file. This communicates with all the robots and keeps track of the messages and state of the world.
- robot_class.py - Implements the robot API of the real world robots for this simulation with communication protocols set using socket programming
- visualization.py - uses pygame to visualize the robots
- user.py - user code written by user to run
- coachbot-simulation.py - launches all the processes and programs correctly, using the config.json
- bootloader.py - launches the user code with the custom coachbot class
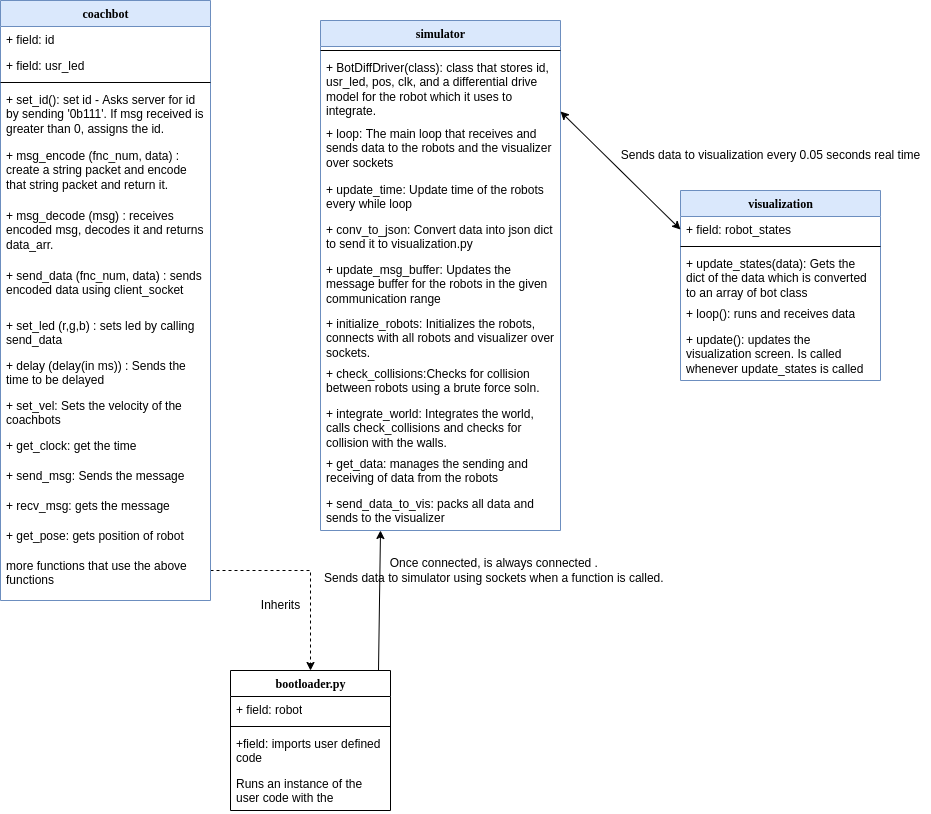
Current program examples
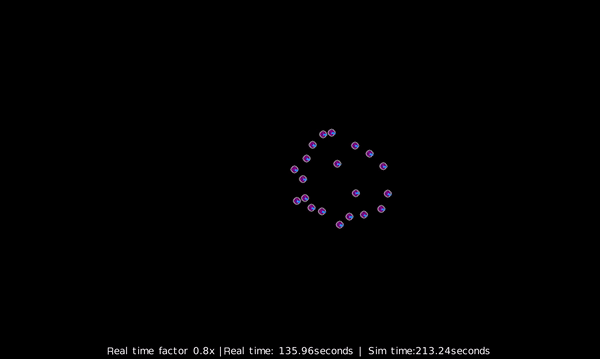
Future work
- Collision detection currently is done through a brute force solution and can create a bottleneck with large number of robots. A more effecient algorithm for collision detection can speed up the simulation.
- The visualization could be a bottleneck with large number of robots. Data is sent from the simulator every 0.05 seconds to the visualizer thread through sockets. It will wait to send all the data. One can increase the time threshold if it is a bottleneck. The visualization code can also be made more efficient by using Sprite and Dirty Sprite and selective update of the screen.
Link for Github
For more, please check this out